Persons import script
In the persons import script, you retrieve the raw personnel data that you'll use in Source mappings to generate your Persons and Contracts objects. It runs during the Source imports process.
Note
Changes to the persons and/or departments import scripts do not have any effect during snapshots. They only have an effect during Source imports.
To get started, Customize the persons import script.
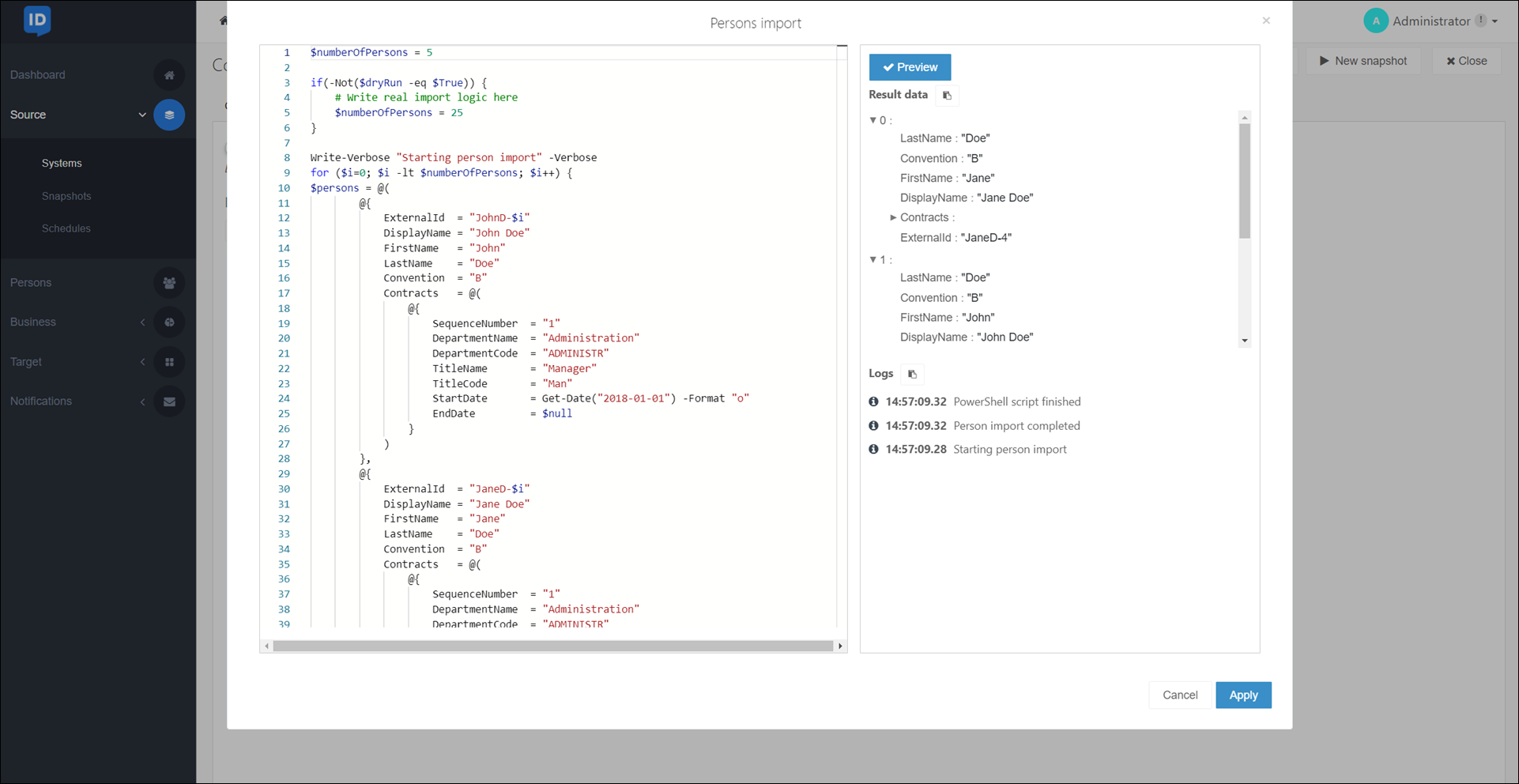
Write your retrieval logic (i.e., query your external HR database) in the if(-Not($dryRun -eq $True)) { }
statement at the beginning of the script. When you are editing and previewing the script, HelloID sets $dryRun to false. When the script runs in production, HelloID sets it to true.
How, and from where you retrieve this information is up to you. For example:
For systems that provide a REST API, use the
Invoke-WebRequest
cmdlet to launch aGET
request.For flat files, use the
Import-CSV
cmdlet to read the data into memory.
Subsequently, using the retrieved raw personnel data, construct a $persons array. Each person is a hash table in this array. The requirements for each person are:
- ExternalId
A unique identifier of your choice (e.g., a GUID)
- DisplayName
A display name for the person (e.g.,
John Doe
). This ensures that persons are recognizable in the raw data.This display name is not used in the source mapping. Instead, the display name of a person in HelloID depends on the
Name.Convention
and otherName
fields included in the default Person schema.- Contracts
An array containing at least one hash table. Hash tables in this array define the person's Contracts.
Note
Keep in mind that after the source mapping, each contract needs to have:
A unique identifier, stored in the
ExternalId
field of a contract.If a Departments import script is used: a
DepartmentCode
, which must correspond to theExternalId
of a department defined in the Departments import script
Although only the ExternalId field is strictly required, it makes sense for contracts to also have at least a start date and a title.
You can add as many key/value pairs to these hash tables as needed, to accommodate whatever data is in your external HR database.
After building your $persons
array, send it to HelloID. Loop through the array and send each person individually via the Write-Output
cmdlet (converted to a JSON string). For example, if you have 1,000 persons, Write-Output
must be called 1,000 times. This is demonstrated in the for
loop, below.
$numberOfPersons = 5
if(-Not($dryRun -eq $True)) {
# Write real import logic here
$numberOfPersons = 25
}
Write-Verbose "Starting person import" -Verbose
for ($i=0; $i -lt $numberOfPersons; $i++) {
$persons = @(
@{
ExternalId = "JohnD-$i"
DisplayName = "John Doe"
FirstName = "John"
LastName = "Doe"
Convention = "B"
Contracts = @(
@{
SequenceNumber = "1"
DepartmentName = "Administration"
DepartmentCode = "ADMINISTR"
TitleName = "Manager"
TitleCode = "Man"
StartDate = Get-Date("2018-01-01") -Format "o"
EndDate = $null
}
)
},
@{
ExternalId = "JaneD-$i"
DisplayName = "Jane Doe"
FirstName = "Jane"
LastName = "Doe"
Convention = "B"
Contracts = @(
@{
SequenceNumber = "1"
DepartmentName = "Administration"
DepartmentCode = "ADMINISTR"
TitleName = "Secretary"
TitleCode = "Sec"
StartDate = Get-Date("2015-03-02") -Format "o"
EndDate = $null
}
)
}
)
foreach ($person in $persons) {
Write-Output $person | ConvertTo-Json
}
}
Write-Verbose "Person import completed" -Verbose