Import permission memberships script
In an Import permission memberships script, you retrieve data from a target system in order to enable HelloID to mark existing permissions, and if used, sub-permissions, as granted in HelloID.
You can add one script for each Permission configuration. They run during the Import target system entitlements process.
Warning
Importing permission memberships can unintentionally revoke sub-permissions.
When permission memberships are imported, any unauthorized sub-permissions are detected by HelloID and as a result, will be revoked during the next enforcement or account update. To prevent this, avoid creating an import script if sub-permissions are enabled in a permission set, so that the sub-permissions remain unmanaged.
To get started, Customize an Import permission memberships script.
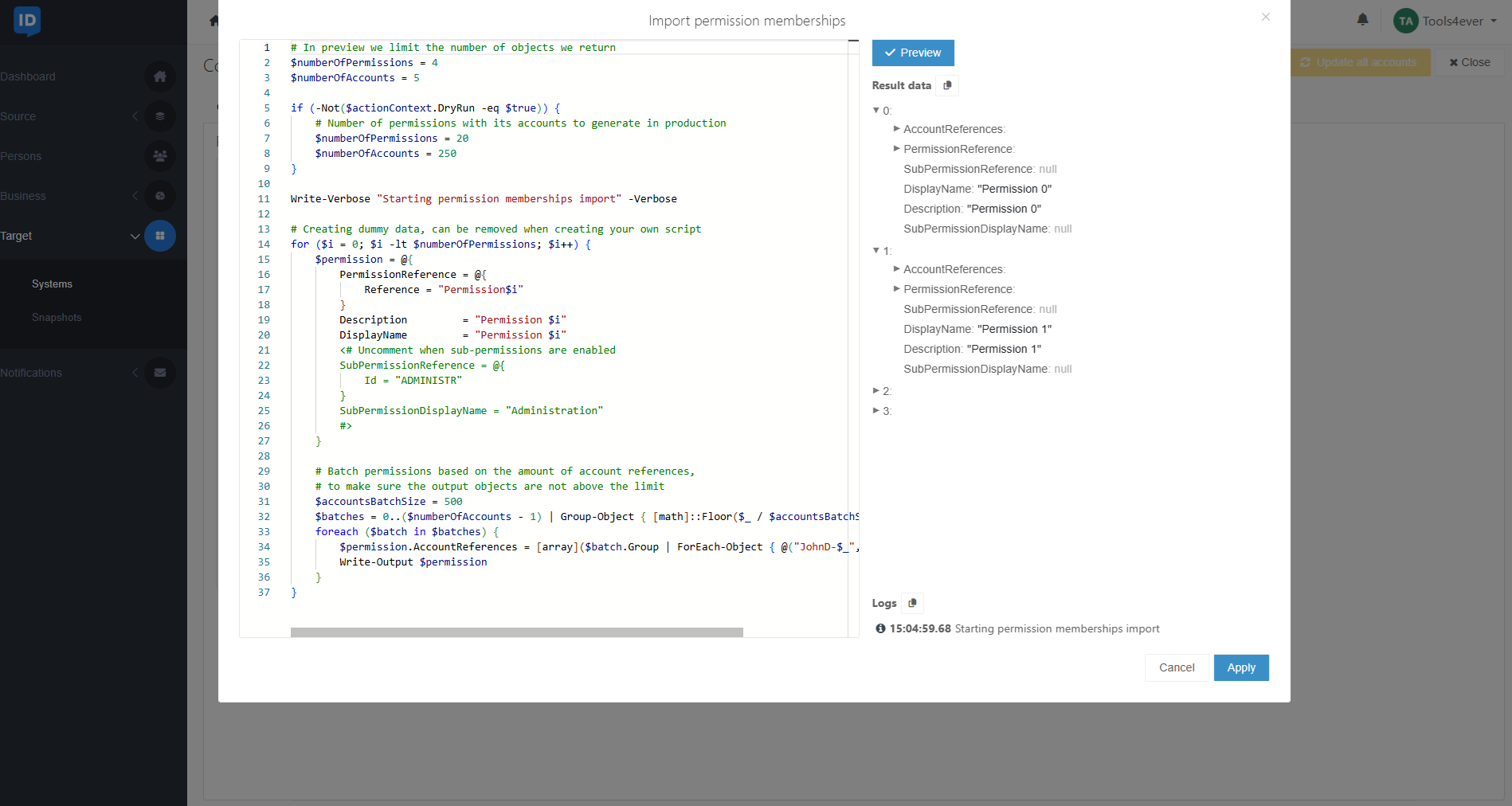
At the beginning of the script, set $numberOfAccounts
and $numberOfPermissions
to the maximum number of accounts resp. permissions to be returned in preview. In preview, this script must finish within 90 seconds.
When you are editing and previewing the script, HelloID sets dryRun
to true
. When the script runs during the Import entitlements process, HelloID sets it to false
.
In the if(-Not($dryRun -eq $True)) { }
statement, set the maximum number of accounts and permissions that can be returned per call to Write-Output
during the Import entitlements process.
Next, write your retrieval logic (i.e., query your PowerShell v2 target system). How, and from where you retrieve this information is up to you.
For systems that provide a REST API, use the
Invoke-WebRequest
cmdlet to launch aGET
request.For flat files, use the
Import-CSV
cmdlet to read the data into memory.
Using the retrieved data, construct $permission
hash tables with the following key-value pairs:
- PermissionReference
A nested hash table defining a reference to a permission.
- Reference
A unique identifier for the permission. This must match the unique identifier of one of the permissions that are configured in this permission configuration.
Example:
PermissionReference = @{ Reference = "Permission$i" }
- Description
A textual description of the permission, for use in reports. Max. 100 characters.
- DisplayName
The display name of the permission, for use in reports. Max. 100 characters.
- Optional: SubPermissionReference
A nested hash table defining a reference to a sub-permission.
- Id
A unique identifier for the sub-permission.
Example:
SubPermissionReference = @{ Id = "ADMINISTR" }
- Optional: SubPermissionDisplayName
The display name of the sub-permission, for use in reports.
- AccountReferences
An array of account references (unique identifiers) assigned to the permission.
Note
SubPermissionReference and SubPermissionDisplayName are required if sub-permissions are enabled in the Permission set.
Return each $permission
to HelloID via Write-Output
.
The AccountReferences array may become too large to send at once when thousands of accounts have the same permission. In that case, process the accounts in batches of 500 accounts.
# In preview we limit the number of objects we return
$numberOfPermissions = 4
$numberOfAccounts = 5
if (-Not($actionContext.DryRun -eq $true)) {
# Number of permissions with its accounts to generate in production
$numberOfPermissions = 20
$numberOfAccounts = 250
}
Write-Verbose "Starting permission memberships import" -Verbose
# Creating dummy data, can be removed when creating your own script
for ($i = 0; $i -lt $numberOfPermissions; $i++) {
$permission = @{
PermissionReference = @{
Reference = "Permission$i"
}
Description = "Permission $i"
DisplayName = "Permission $i"
<# Uncomment when sub-permissions are enabled
SubPermissionReference = @{
Id = "ADMINISTR"
}
SubPermissionDisplayName = "Administration"
#>
}
# Batch permissions based on the amount of account references,
# to make sure the output objects are not above the limit
$accountsBatchSize = 500
$batches = 0..($numberOfAccounts - 1) | Group-Object { [math]::Floor($_ / $accountsBatchSize ) }
foreach ($batch in $batches) {
$permission.AccountReferences = [array]($batch.Group | ForEach-Object { @("JohnD-$_", "JaneD-$_") })
Write-Output $permission
}
}