PowerShell data sources
Use PowerShell data sources to populate Form elements with the output of a custom PowerShell script. They are the most commonly used type of data source, and the most powerful.
To get started, Add a PowerShell data source.
PowerShell data sources use native PowerShell logging.
Write-Information "Information"
Write-Host "Equivalent to Write-Information"
Write-Error "An error"
Write-Warning "A warning"
Write-Verbose -Verbose "Verbose requires the -Verbose flag"
Write-Debug -Debug "Debug requires the -Debug flag"
Tip
HelloID interprets Write-Error
messages as task failure, in which case the task execution will be labeled Failed in the data source logs.
When testing your script, you can preview log messages on the Received Logs tab. To view them in production, View logs. Alternatively, if the data source is in a delegated form, View data source logs.
Control which PowerShell log levels are shown on the Received Logs tab using the $VerbosePreference
, $InformationPreference
, and $WarningPreference
variables. Levels set to Continue
will be shown. Levels set to SilentlyContinue
will not.
When you create, edit, or delete a PowerShell data source, the action is automatically logged in the Audit logs.
Writing custom messages into audit logs from PowerShell data sources is not currently supported.
Email sends are not currently supported in PowerShell data sources.
A PowerShell data source should return one or multiple hashtables via the Write-Output cmdlet, depending on the type of form element it's connected to:
Single option form elements
Single option form elements (e.g., text input) expect a single hashtable. For example:
# Text Input form element - PowerShell datasource example
$VerbosePreference = "SilentlyContinue"
$InformationPreference = "Continue"
$WarningPreference = "Continue"
try {
Write-Verbose "Querying data"
# Execute action to query data
# Example: Get data from API
# $results = Invoke-RestMethod -URI "<DataEndpoint>" -Method GET -UseBasicParsing -ErrorAction Stop
$result = @()
$result += (@{
text="Text 1";
})
Write-Information "Successfully queried data. Result count: $(($results | Measure-Object).Count)"
$returnObject = @{
TextInputText = $result.text
}
Write-Output $returnObject
} catch {
$ex = $PSItem
Write-Verbose "Error at Line '$($ex.InvocationInfo.ScriptLineNumber)': $($ex.InvocationInfo.Line). Error: $($ex.Exception.Message)"
Write-Error "Error querying data. Error Message: $($_ex.Exception.Message)"
}
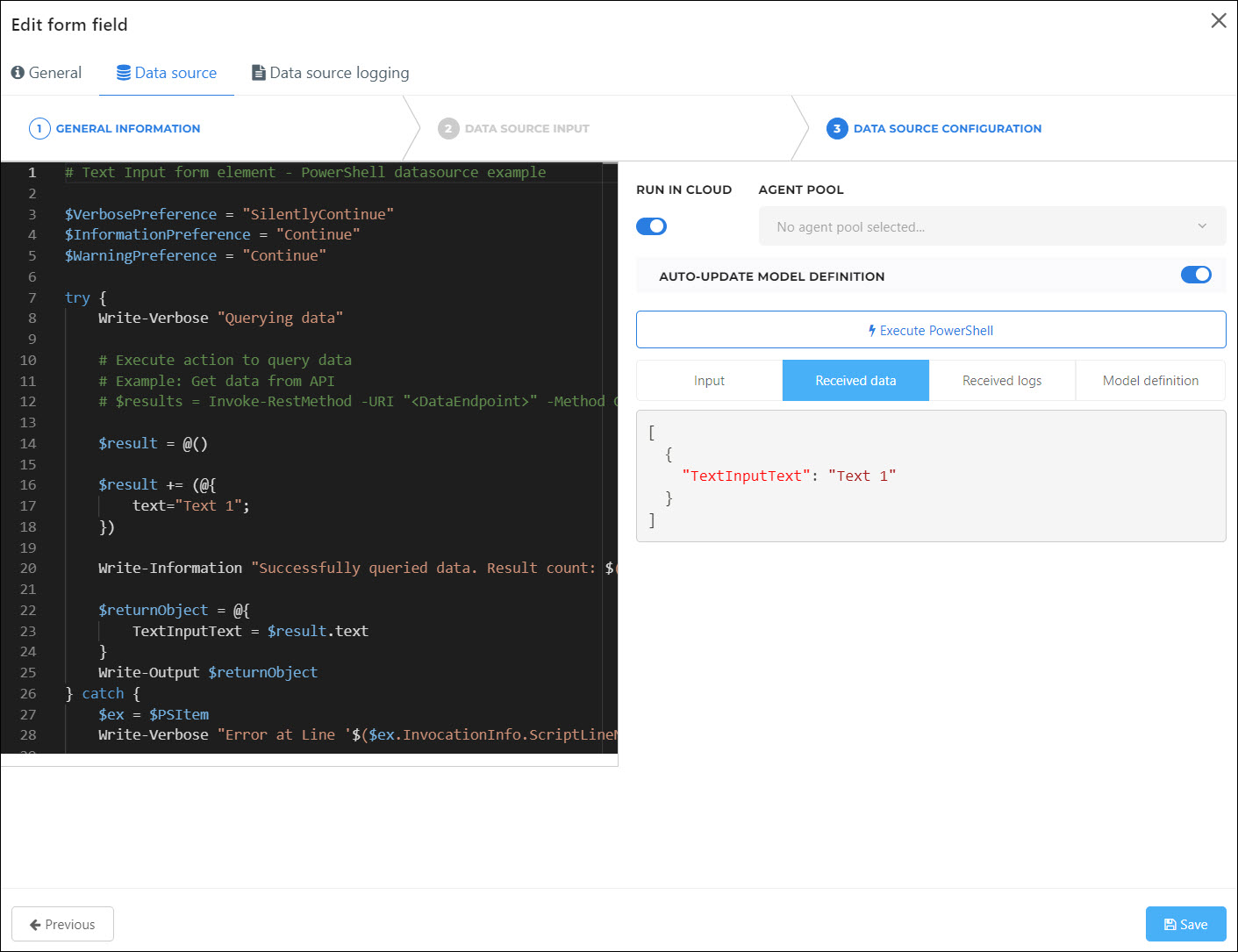
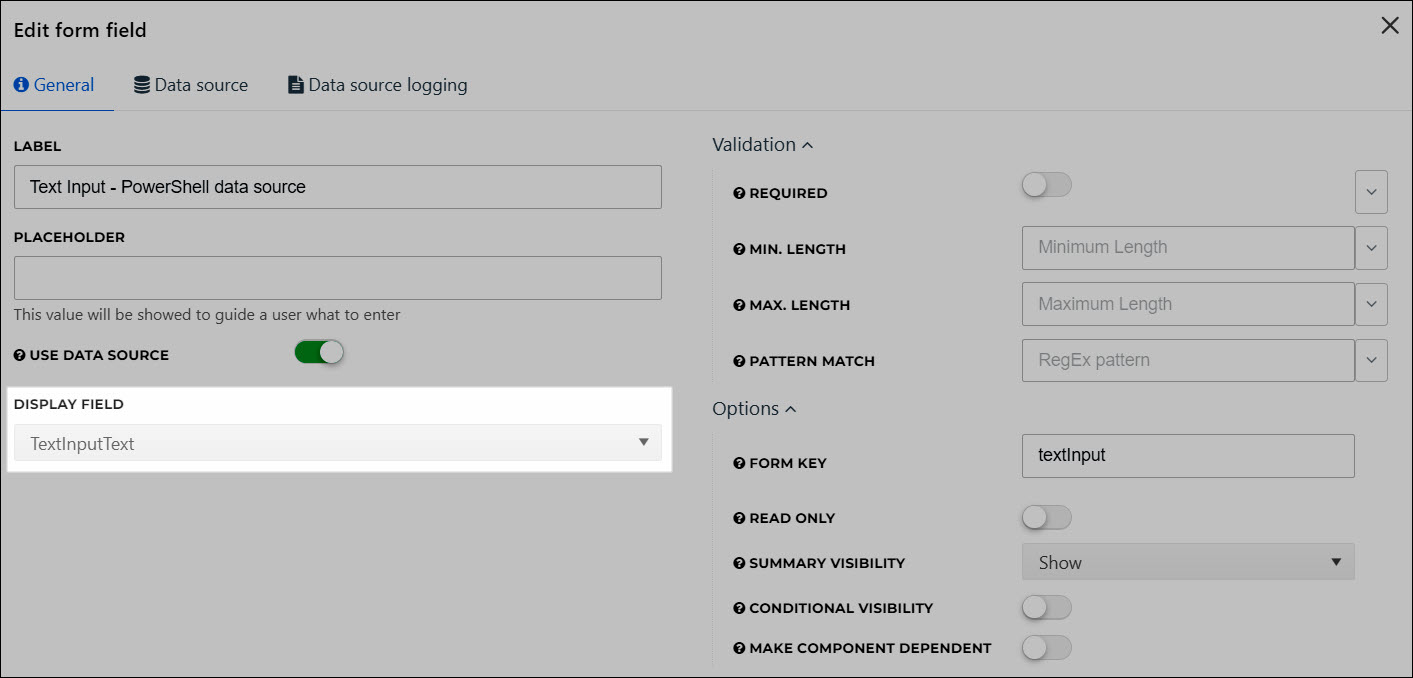

Multiple option form elements
Multiple option form elements (e.g., grid, dropdown, dual list) expect an array or list of hashtables. For example:
# Dropdown form element - PowerShell datasource example
$VerbosePreference = "SilentlyContinue"
$InformationPreference = "Continue"
$WarningPreference = "Continue"
try {
Write-Verbose "Querying data"
# Execute action to query data
# Example: Get data from API
# $results = Invoke-RestMethod -URI "<DataEndpoint>" -Method GET -UseBasicParsing -ErrorAction Stop
$results = @()
$results += (@{
value="DataValue1";
text="Text 1";
})
$results += (@{
value="DataValue2";
text="Text 2";
})
$results += (@{
value="DataValue3";
text="Text 3";
})
# Example: Sort results in specific order
# $results = $results | Sort-Object -Property "<UniqueKey>"
Write-Information "Successfully queried data. Result count: $(($results | Measure-Object).Count)"
if (($results | Measure-Object).Count -gt 0) {
foreach ($result in $results) {
$returnObject = @{
DropDownValue = $result.value
DropDownText = $result.text
}
Write-Output $returnObject
}
}
} catch {
$ex = $PSItem
Write-Verbose "Error at Line '$($ex.InvocationInfo.ScriptLineNumber)': $($ex.InvocationInfo.Line). Error: $($ex.Exception.Message)"
Write-Error "Error querying data. Error Message: $($_ex.Exception.Message)"
}
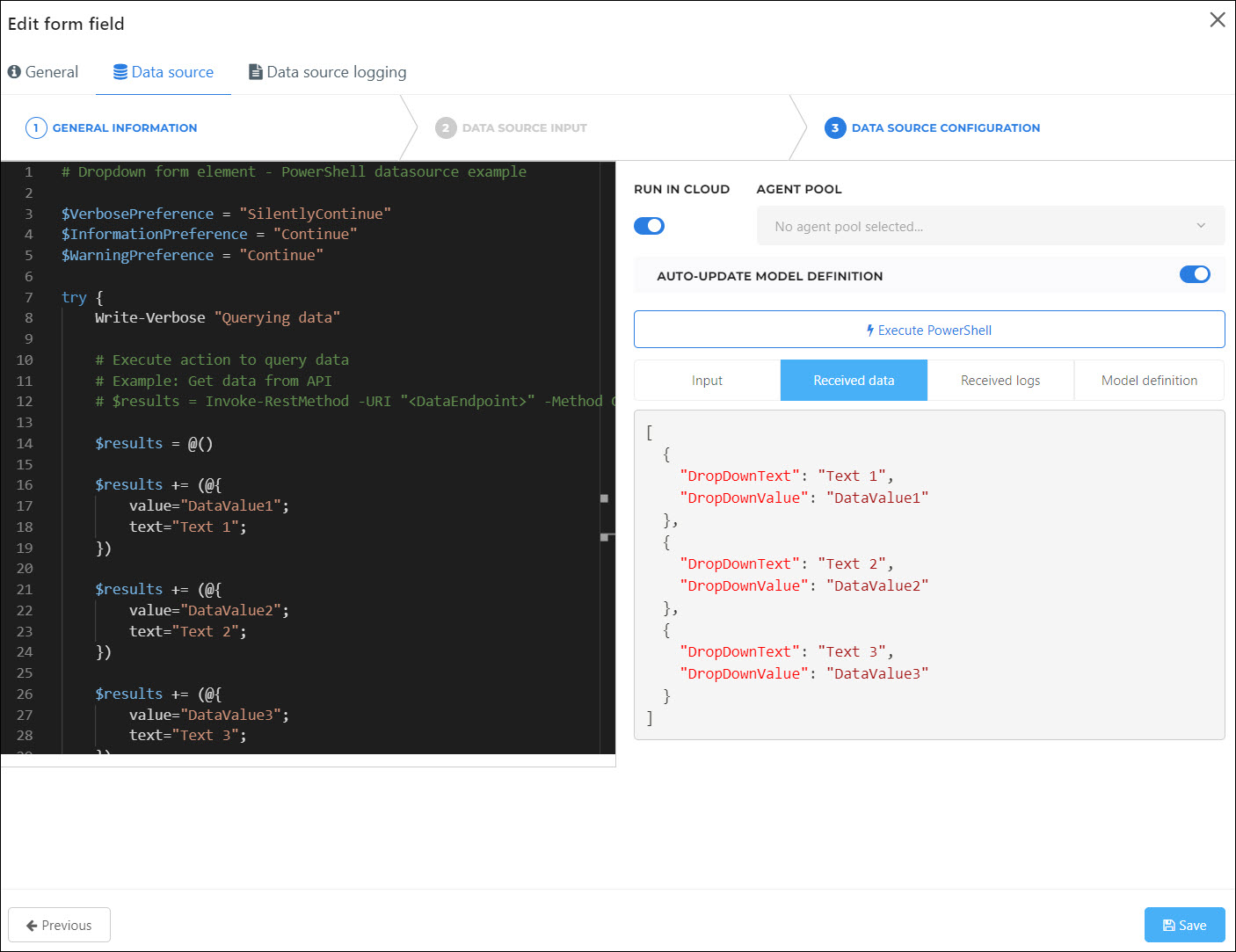
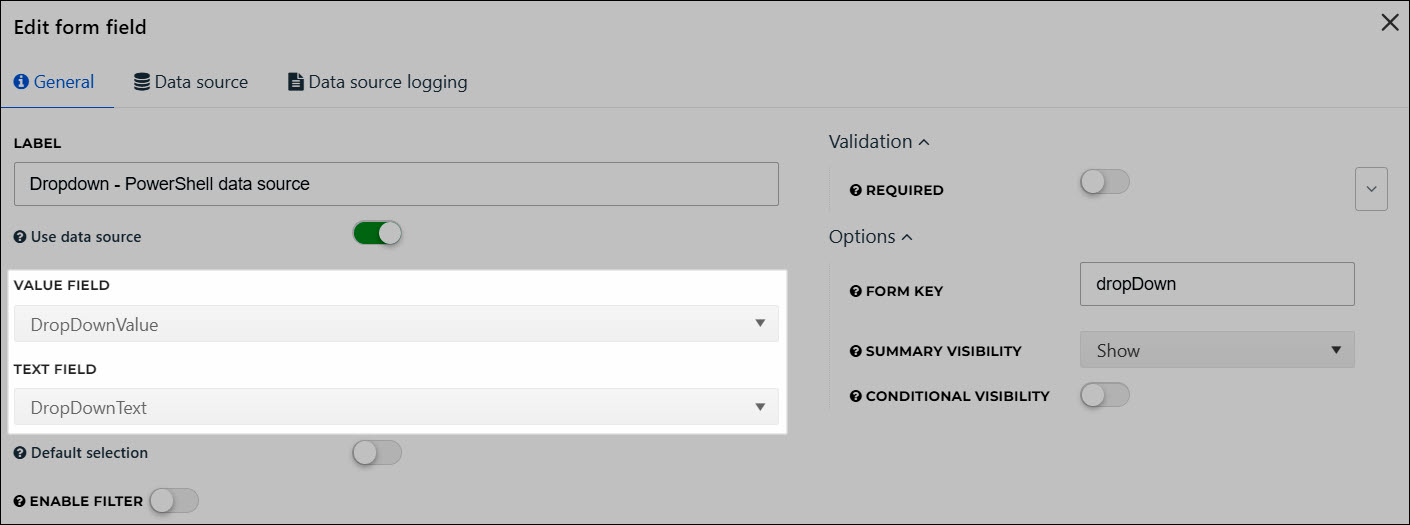
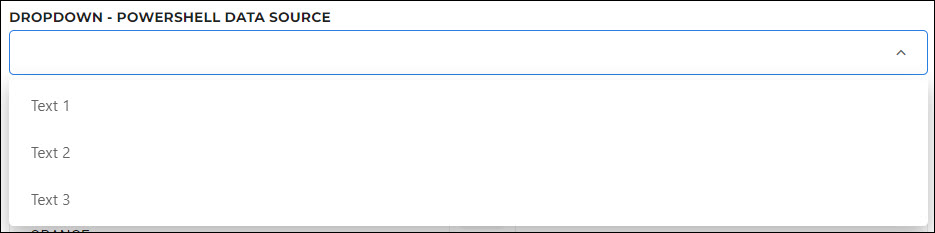