Account data import script
In the account data import script, you retrieve account data so that HelloID can correlate existing accounts with persons and mark account access as granted if the account is enabled. This script runs during the Import entitlements process.
To get started, Customize the account data import script.
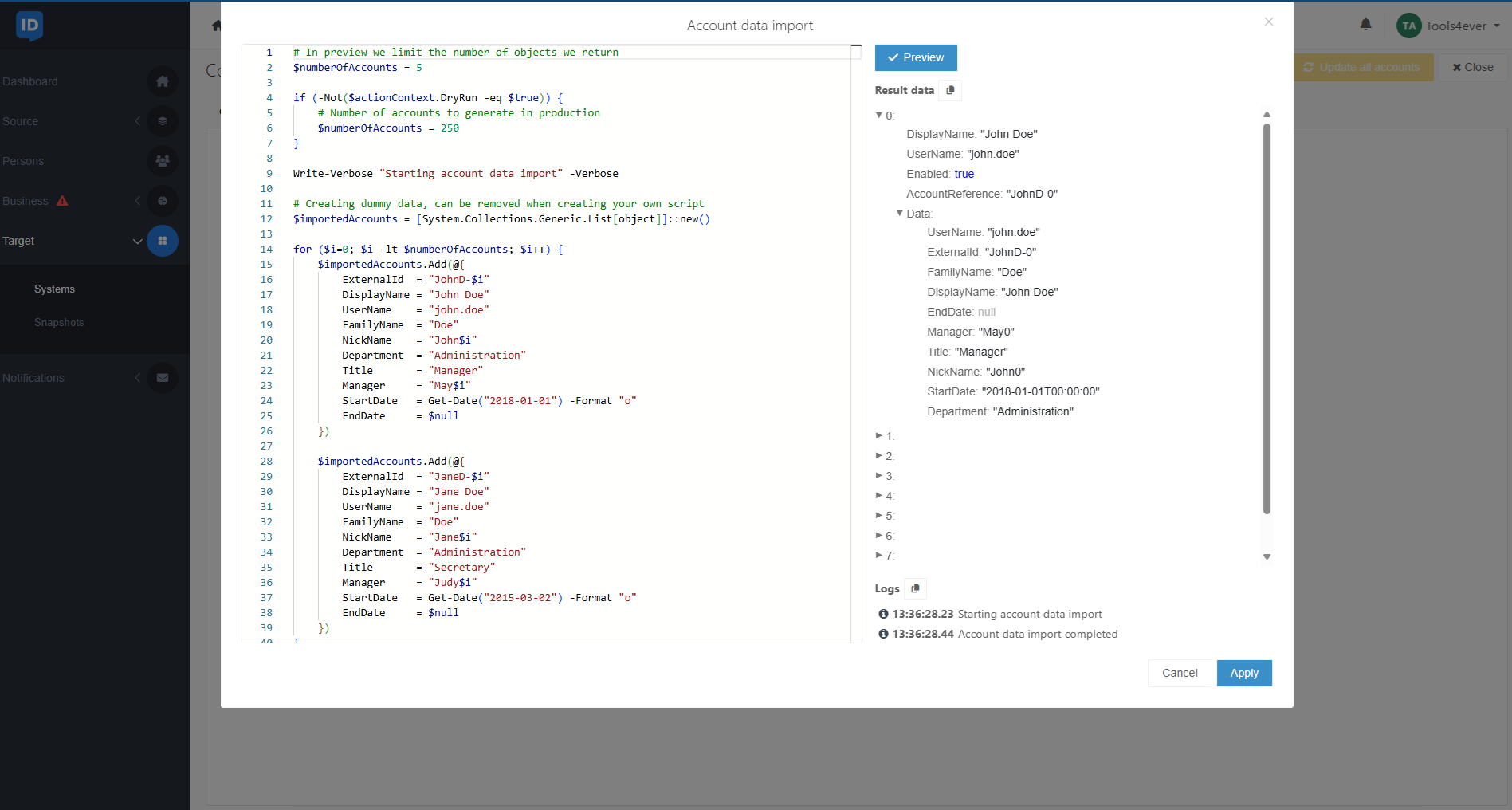
At the beginning of the script, set $numberOfAccounts
to the maximum number of accounts that can be returned in preview. Preview calls are limited to 90 seconds.
When you are editing and previewing the script, HelloID the $dryRun variable to true
. When the script runs during the Import entitlements process, HelloID sets it to false
.
Next, write your retrieval logic (i.e., query your PowerShell v2 target system). How, and from where you retrieve this information is up to you. For example:
For systems that provide a REST API, use the
Invoke-WebRequest
cmdlet to launch aGET
request.For flat files, use the
Import-CSV
cmdlet to read the data into memory.
Send each retrieved account individually to HelloID via the Write-Output
cmdlet. For example, if you have 1,000 accounts, Write-Output
must be called 1,000 times.
Each account must be a hash table with the following fields:
- AccountReference
The account's unique identifier
- DisplayName
The display name of the account, for use in reports. Max. 100 characters.
- UserName
The user name of the account, for use in reports. Max. 100 characters.
- Enabled
Whether the account is enabled:
$true
or$false
- Data
A hash table containing the data to save to the corresponding person in HelloID if the account gets correlated.
Requirements:
Since only mapped target account fields can be saved to a person in HelloID, the imported data needs to be mapped to the mapped target account fields. A list of these fields is available in the script through
$actionContext.ImportFields
.The data must include the correlation attribute, even if its value is null or empty.
Return any shared account fields to make them available to dependent systems. Normally, this would happen when an account is created or updated in the shared system, but when an account entitlement is imported, these actions are not performed.
# In preview we limit the number of objects we return
$numberOfAccounts = 5
if (-Not($actionContext.DryRun -eq $true)) {
# Number of accounts to generate in production
$numberOfAccounts = 250
}
Write-Verbose "Starting account data import" -Verbose
# Creating dummy data, can be removed when creating your own script
$importedAccounts = [System.Collections.Generic.List[object]]::new()
for ($i=0; $i -lt $numberOfAccounts; $i++) {
$importedAccounts.Add(@{
ExternalId = "JohnD-$i"
DisplayName = "John Doe"
UserName = "john.doe"
FamilyName = "Doe"
NickName = "John$i"
Department = "Administration"
Title = "Manager"
Manager = "May$i"
StartDate = Get-Date("2018-01-01") -Format "o"
EndDate = $null
})
$importedAccounts.Add(@{
ExternalId = "JaneD-$i"
DisplayName = "Jane Doe"
UserName = "jane.doe"
FamilyName = "Doe"
NickName = "Jane$i"
Department = "Administration"
Title = "Secretary"
Manager = "Judy$i"
StartDate = Get-Date("2015-03-02") -Format "o"
EndDate = $null
})
}
# Map the imported data to the account field mappings
foreach ($importedAccount in $importedAccounts)
{
$data = @{}
foreach ($field in $actionContext.ImportFields) {
$data[$field] = $importedAccount[$field]
}
# Return the result
Write-Output @{
AccountReference = $importedAccount.ExternalId
DisplayName = $importedAccount.DisplayName
UserName = $importedAccount.UserName
Enabled = $true
Data = $data
}
}
Write-Verbose "Account data import completed" -Verbose